Page Controls
A page control displays a horizontal series of dots, each of which represents a page or screen in an app. Although a page control doesn’t manage the display of content pages, you can write code that lets users navigate between pages by tapping the control. You can see examples of page controls in Weather—when you set more than one location—and in the summary, chart, and news view in Stocks (in portrait orientation). Typically, a page control is used with another view—such as a scroll view—that manages the pages and handles scrolling, panning, and zooming of the content. In this scenario, the scroll view usually uses paging mode to display the content, which is divided into separate views or into separate areas of one view.
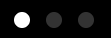
Purpose. Page controls allow users to:
Have a visual indication of which page is currently displayed
Navigate between pages in an app
Implementation. Page controls are implemented in the UIPageControl
class and discussed in the UIPageControl Class Reference.
Configuration. Configure page controls in Interface Builder, in the Page Control section of the Attributes Inspector. A few configurations cannot be made through the Attributes Inspector, so you must make them programmatically. You can set other configurations programmatically, too, if you prefer.
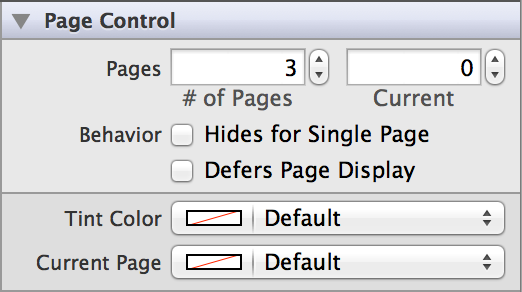
Content of Page Controls
To specify the number of dots the page control should display, you can use the the # of Pages (numberOfPages
) field in the Attributes Inspector. Note that when you create a page control in Interface Builder, the default number of dots is 3; when you create a page control programmatically, the default number of dots is 0.
Use the Current (currentPage
) field to specify the currently selected page. Note that page index begins at 0 instead of 1, so the maximum index of the currently selected page is one less than the total number of pages.
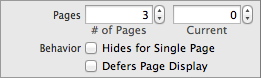
Behavior of Page Controls
Page controls need a delegate to handle user interaction. A page control doesn't automatically stay synchronized with the currently open page—or let users tap the control to transition between pages—unless you enable these actions in your app. To ensure that a page control's current-page dot corresponds to the page that is currently open in the scroll view, implement the UIScrollViewDelegate
protocol in your view controller. Then, update the page control in the scrollViewDidScroll:
delegate method and set the page control's currentPage
property to the current page.
A page control sends the UIControlEventValueChanged
event when the user taps it. You can respond to this event by performing some corresponding action in your app, such as transitioning to a different page in the scroll view. You register the target-action methods for a page control as shown below.
[self.myPageControl addTarget:self
action:@selector(myAction:)
forControlEvents:UIControlEventValueChanged];
Alternatively, you can Control-drag the page control’s Value Changed event from the Connections Inspector to the action method. For more information, see Target-Action Mechanism.
By default, a page control displays one dot when an app contains only one page. To set the page control to display no dots when there is only one page, select the Hides for Single Page (hidesForSinglePage
) checkbox in the Attributes Inspector.
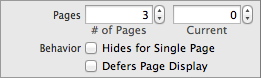
You can also choose to delay updating the page control’s display when the current page changes. If the Defers Page Display (defersCurrentPageDisplay
) box is enabled, when the user clicks the control to go to a new page, the class defers updating the page control until it calls updateCurrentPageDisplay
. This behavior is off by default, which means the page indicator updates immediately.
Appearance of Page Controls
You can customize the appearance of a page control by setting the properties depicted below.
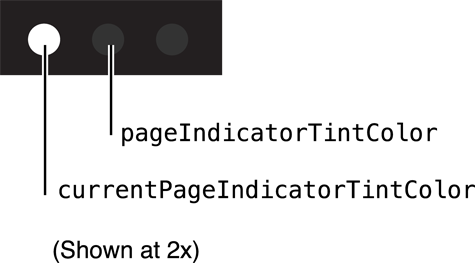
To customize the appearance of all page controls in your app, use the appearance proxy (for example, [UIPageControl appearance]
). For more information about appearance proxies, see Appearance Proxies.
Tint Color
The only way to customize the appearance of a page control is by setting custom tints for the dots representing each page. The Current Page (currentPageIndicatorTintColor
) field affects the color of the dot representing the currently displayed page, and the Tint Color (pageIndicatorTintColor
) field affects the color of the dots representing every other page. The default color is white for the current page dot, and translucent gray for the other page dots.

If you want your custom colors to be translucent, you must specify a color with an alpha value of less than 1.0. This must be done programmatically, as in the following example:
self.myPageControl.currentPageIndicatorTintColor = [UIColor colorWithRed:0.0 green:0.0 blue:1.0 alpha:0.5];
self.myPageControl.pageIndicatorTintColor = [UIColor colorWithRed:1.0 green:0.0 blue:0.0 alpha:0.5];
Using Auto Layout with Page Controls
You can create auto layout constraints between a page control and other user interface elements. You can create any type of constraint for a page control besides a baseline constraint.
To keep a page control centered onscreen, you can use auto layout to pin a page control to its superview or align it with other elements. Typically, you leave space for a page control at the bottom of the screen, below the view that displays the pages.
For general information about using auto layout with iOS controls, see Using Auto Layout with Controls.
Making Page Controls Accessible
Page controls are accessible by default. The default accessibility traits for a page control are Updates Frequently and User Interaction Enabled. The Updates Frequently accessibility trait means that the page control doesn't send update notifications when its state changes. This trait tells an assistive app that it should poll for changes in the page control when necessary.
When the user interacts with a page control, VoiceOver speaks "page x of y" where x is the current page and y is the total number of pages.
For general information about making iOS controls accessible, see Making Controls Accessible.
Internationalizing Page Controls
Page controls have no special properties related to internationalization.
For more information, see Internationalization Programming Topics.
Debugging Page Controls
When debugging issues with page controls, watch for this common pitfall:
Selecting an out of range current page. If you try to set a page control’s current page to be higher than the index of the last page, it will be set equal to the index of the last page. If you try to set a page control’s current page to be lower than 0, it will be set to 0.
Elements Similar to a Page Control
The following elements provide similar functionality to a page control:
Scroll View. A class that supports a page-by-page scrolling experience in addition to panning and zooming of content. For more information, see Scroll Views.
Page View Controller. A class that displays multiple content views in a book-like format and enables animated transitions between pages. For more information, see UIPageViewController Class Reference.
Copyright © 2014 Apple Inc. All rights reserved. Terms of Use | Privacy Policy | Updated: 2013-12-16