Web Views
A web view is a region that can display rich HTML content (shown here between the navigation bar and toolbar in Mail on iPhone).
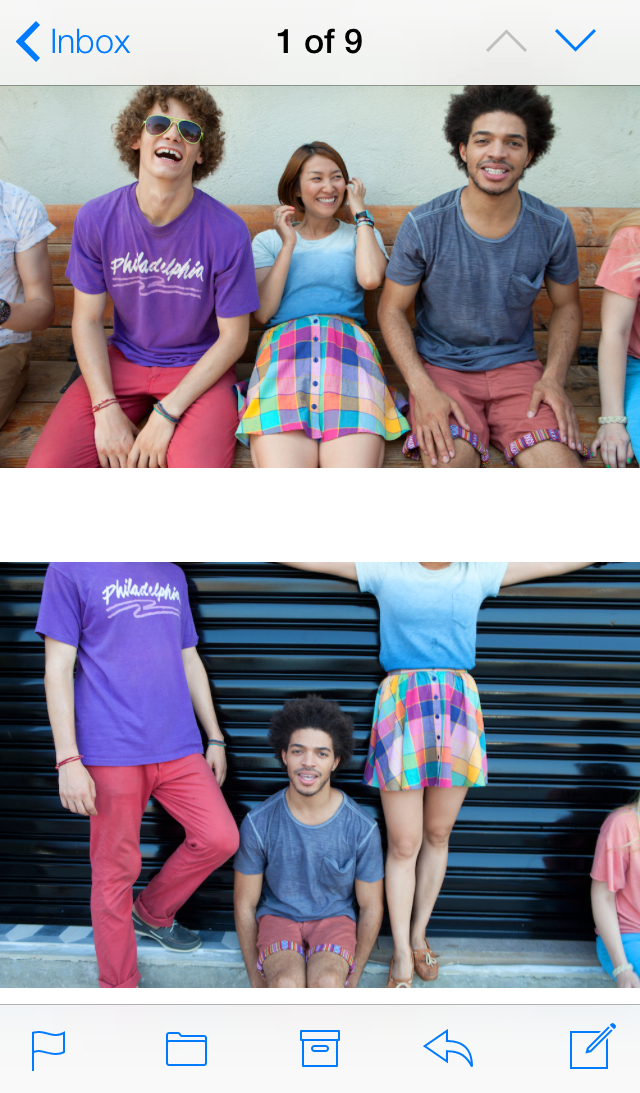
Purpose. Web views allow users to:
View web content within an app
Implementation. Web views are implemented in the UIWebView
class and discussed in the UIWebView Class Reference.
Configuration. Configure web views in Interface Builder, in the Web View section of the Attributes Inspector. A few configurations cannot be made through the Attributes Inspector, so you must make them programmatically. You can set other configurations programmatically, too, if you prefer.
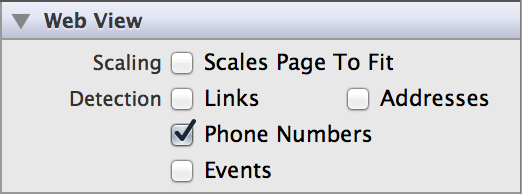
Content of Web Views (Programmatic)
To get your web view to display content, you simply create a UIWebView
object, attach it to a window, and send it a request to load web content. Use the loadRequest:
method to begin loading web content, the stopLoading
method to stop loading, and the loading
property to find out if a web view is in the process of loading. You can create the web view object in code or in Interface Builder, but you can load content in code only.
[self.myWebView loadRequest:[NSURLRequest requestWithURL:[NSURL URLWithString:@"http://www.apple.com/"]]];
Behavior of Web Views
You can set your web view to automatically scale web content to fit on the screen of the user’s device. By default, this behavior is disabled, but you can enable it by checking the Scales Page To Fit (scalesPageToFit
) box in Attributes Inspector. Enabling this property also allows the user to zoom in and out in the web view.
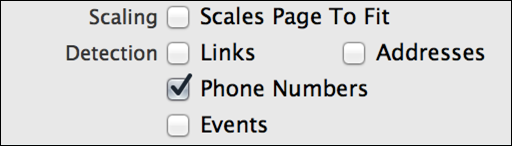
A web view is capable of recognizing when web text is formatted as a link, address, phone number, or event. If you enable the corresponding Detection (dataDetectorTypes
) checkboxes, users will be able to trigger the appropriate action for each type of text by clicking it in the web view. For example, they will be able to call a phone number or add an event to their calendar.
Appearance of Web Views
You cannot customize the appearance of a web view.
Using Auto Layout with Web Views
You can create Auto Layout constraints between a web view and other user interface elements. You can create any type of constraint for a web view besides a baseline constraint.
You generally want the web view to fill the full width of your screen. To ensure that this happens correctly on all devices and orientations, you can create “Leading Space to Superview” and “Trailing Space to Superview” constraints, and set both values equal to 0. This will ensure that the web view remains pinned to the edges of the device screen.
For general information about using Auto Layout with iOS views, see Using Auto Layout with Views.
Making Web Views Accessible
Web views are accessible by default. The default accessibility trait for a web view is "User Interaction Enabled.”
For general information about making iOS views accessible, see Making Views Accessible.
Internationalizing Web Views
Web views have no special properties related to internationalization.
For more information, see Internationalization Programming Topics.
Debugging Web Views
When debugging issues with web views, watch for these common pitfalls:
Placing a web view inside of a scroll view. Web views handle their own scrolling. You should not embed web view objects in scroll views. If you do so, unexpected behavior can result because touch events for the two objects can be mixed up and wrongly handled.
Not testing web content size. Web content comes in a variety of sizes, and it may be difficult to view content that is too large or too small for a device screen. Enable the
scalesPageToFit
property to allow users to zoom in or out if you anticipate that this might be the case for your app.Not having a valid Internet connection. Since web views rely entirely on the Internet, a working connection is essential to loading web view content. Slow or disabled connections may make it appear as if your web view is not functioning properly when the actual problem is with the connection.
Elements Similar to a Web View
The following element provides similar functionality to a web view:
Scroll View. Use a scroll view for scrollable content. For more information, see Scroll Views.
Copyright © 2014 Apple Inc. All rights reserved. Terms of Use | Privacy Policy | Updated: 2013-12-16